News
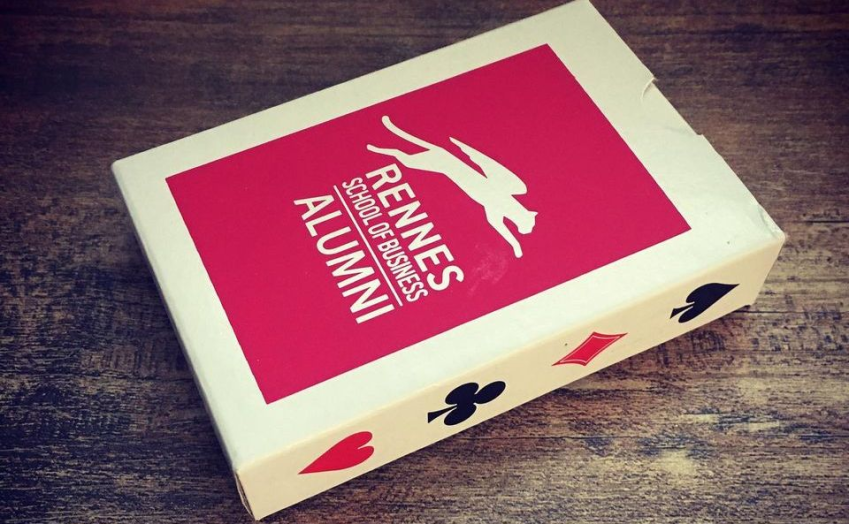
Nouveau partenariat BDE - Alumni
L'association Rennes SB Alumni est très heureuse d'avoir signé récemment un partenariat avec le BDE Krak Rennes, dans l'objectif de créer du lien entre les étudiants et les diplômés.
Cette initiative est soutenue côté Alumni par 2 membres du Comité (Alice CAILLOSSE - IBPM 8 et Rémi RIVAS - PGE 17). Les étudiants (PGE 30) déjà impliqués sont Nicolas ANCELLIN, Amelys AMGHAR, Thomas SCHOTT, Maha TIRABOSCHI et Jade DIEP. Ils recrutent actuellement une équipe qui composera le "Club Alumni" au sein du BDE pour l'année à venir.
1ère réalisation concrète : les étudiants ayant participé à la semaine d'intégration organisée par le BDE ont reçu un jeu de carte aux couleurs de l'association Alumni dans leurs "Welcome Packs".
Pour tout savoir sur ce partenariat et ses réalisations, vous pouvez suivre la page Facebook du Club : https://www.facebook.com/clubalumnirsb/.
Aucun commentaire
Vous devez être connecté pour laisser un commentaire. Connectez-vous.